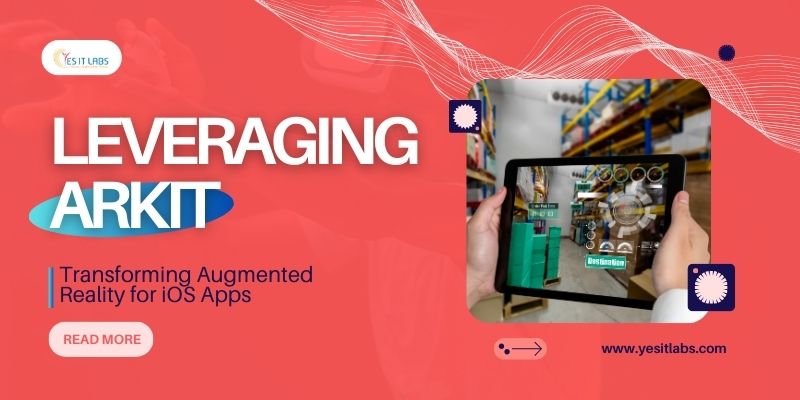

Leveraging ARKit: Transforming Augmented Reality for iOS Apps
Augmented Reality (AR) has become a cornerstone of modern technology, revolutionizing the way users interact with digital environments. At the heart of this transformation is Apple’s ARKit, a robust framework for building immersive AR experiences on iOS devices. This article delves into the unique capabilities of ARKit, offering insights into its features, real-world applications, and how developers can harness its potential for crafting innovative iOS applications.
Understanding ARKit
ARKit is Apple’s proprietary framework that combines advanced hardware and software capabilities to enable augmented reality on iOS devices. It leverages the camera, motion sensors, and sophisticated algorithms to seamlessly blend virtual objects with the real world. For companies offering iOS application development services, ARKit is a game-changing tool that simplifies AR app creation while ensuring optimal performance on Apple devices.
Core Features of ARKit
- Advanced Motion Tracking
ARKit utilizes Visual Inertial Odometry (VIO), combining data from the device’s motion sensors and camera. This ensures virtual objects remain stable and fixed within the environment, even as the user moves around. - Scene Analysis and Object Recognition
- Plane Detection: ARKit detects flat surfaces like floors and walls, allowing accurate placement of digital elements.
- Object Recognition: It can identify and respond to specific real-world objects, enabling enhanced interactivity.
- Environmental Blending
With light estimation, ARKit adjusts virtual objects’ brightness and shadows based on real-world lighting conditions, creating a seamless visual blend. - Face and Body Tracking
Using the TrueDepth camera, ARKit maps facial expressions and tracks up to three faces at once. For fitness and gaming apps, its body tracking capabilities offer full-body pose detection. - LiDAR and Depth Perception
On LiDAR-equipped devices, ARKit uses precise depth sensing to enhance occlusion and scene understanding, enabling richer AR interactions.
For businesses offering iOS apps development services, these features unlock the potential for creating sophisticated AR applications tailored to diverse industries.
Steps to Build an AR App Using ARKit
- Setting Up the Environment
Start by integrating ARKit and SceneKit frameworks into your Xcode project. Configure the AR session with an appropriate configuration, such as ARWorldTrackingConfiguration, to track device movements and detect planes. - Scene Understanding and Object Placement
Implement plane detection and anchors to accurately position virtual objects in the environment. Customize object interactions using gestures like taps, rotations, and pinches. - Rendering with RealityKit or SceneKit
Use RealityKit for its advanced rendering and physics capabilities or SceneKit for easier implementation. For custom rendering, leverage the Metal framework for complete control.
By partnering with a mobile software development company experienced in ARKit, you can ensure your app utilizes these capabilities effectively, delivering a smooth user experience.
Expert Tips for ARKit Development
- Optimize Performance for Seamless Experiences
- Reduce texture sizes and optimize 3D models to enhance rendering speeds.
- Implement occlusion to avoid rendering objects outside the user’s view.
- Utilize Persistent AR Anchors
Save AR session data using ARWorldMap or ARKit’s collaborative sessions to let users return to their AR setups later. - Leverage LiDAR for Enhanced Accuracy
For devices with LiDAR, use depth maps to improve object placement and environmental understanding, especially in complex scenes. - Train Custom Models for Object Recognition
Integrate machine learning models created with CreateML to identify specific objects, enabling advanced AR functionalities tailored to your app’s purpose. - Experiment with Reality Composer
Reality Composer, a drag-and-drop AR creation tool, simplifies the prototyping and implementation of AR experiences, making it perfect for testing concepts rapidly.
These tips can help businesses hire iOS app developers who are adept at ARKit to build applications that stand out in competitive markets.
Applications of ARKit Across Industries
- Retail and E-commerce
- Virtual Try-Ons: Apps like Warby Parker let users try on glasses virtually, improving shopping experiences.
- Furniture Placement: IKEA Place enables users to visualize furniture in their space before purchasing.
- Gaming and Entertainment
- Pokémon GO: With ARKit integration, the app places Pokémon in real-world environments for engaging gameplay.
- Angry Birds AR: Offers interactive gaming by merging virtual elements with the user’s surroundings.
- Education and Training
- Anatomy 4D: Delivers interactive 3D models for medical students to explore human anatomy.
- Measure: Apple’s built-in app measures real-world dimensions using ARKit.
- Industrial and Business Solutions
ARKit powers simulations, design visualizations, and maintenance guides, showcasing its versatility for enterprise use.
For businesses offering iOS apps development services, these applications illustrate the potential of ARKit to cater to diverse user needs and industries.
Why Choose ARKit for AR Development?
- Native Integration
ARKit is deeply integrated with iOS hardware and software, ensuring consistent performance and a polished user experience. - Comprehensive Development Tools
Apple provides a wide range of APIs and libraries that streamline the development process, allowing developers to focus on creativity and innovation. - Scalability
ARKit’s capabilities scale seamlessly across Apple devices, from iPhones to iPads, enabling broad user reach. - Large User Base
With millions of iOS users worldwide, ARKit apps have immense potential to capture a diverse and engaged audience.
By partnering with a reliable mobile software development company, you can maximize these benefits, ensuring your app is not only functional but also engaging and innovative.
Conclusion
ARKit is a powerful framework that bridges the gap between the real and virtual worlds, enabling developers to craft unique and immersive AR experiences. Whether you’re creating a game, an educational app, or an enterprise solution, ARKit’s advanced features provide endless opportunities for innovation.
For businesses exploring iOS application development services, leveraging ARKit is a strategic choice. By hiring skilled developers or collaborating with experienced teams, you can build AR apps that captivate users and drive success in the competitive iOS market.
Tags: app development company in usa, app development company usa, ARKit, ARKit Tool, Augmented Reality for iOS Apps, best mobile app development company in usa, custom app development company, custom ios app development, custom iphone app development company, hire ios app developer, hire iOS app developers, hire iOS developer, hire ios developers, hire iphone app developer, iOS app development Company, ios app development services, ios development company, ios mobile app development company, iphone app developers, iphone app development company, iphone app development services, mobile app development companies, mobile app development companies in usa, mobile app development company in usa, mobile app development company usa, mobile software development companyLatest Resources
ChatGPT and DeepSeek: Which AI Tool Delivers Better User Experience?
January 29, 2025
Top Frameworks for Cross-Platform App Development in 2025
January 22, 2025
A Guide to Types of Artificial Intelligence (AI)
January 14, 2025
Key Benefits of React Native App Development
January 7, 2025
Leveraging AI in Startup Software Development: Trends and Tips
December 30, 2024